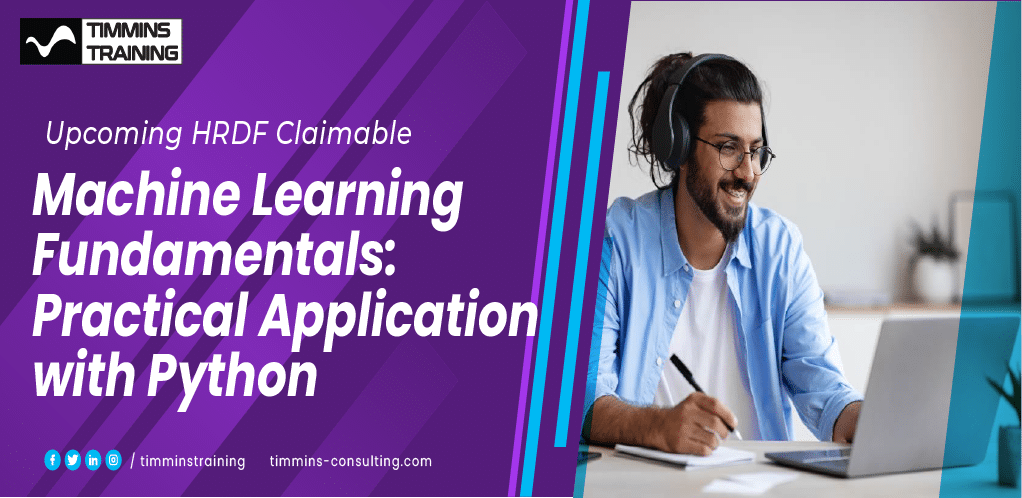
Machine Learning Fundamentals: Practical Application with Python – Part 2
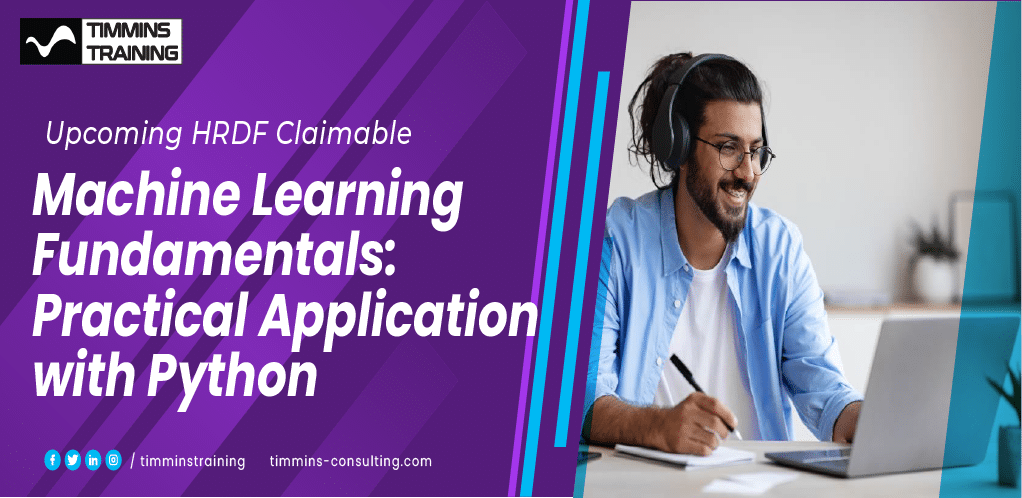
HRD Corp Course Registration No:
10001321038
Duration:
16 hours
Course Description
This course provides a comprehensive understanding of machine learning, covering key topics such as theory, problem formulation, and algorithms. Participants will learn to apply machine learning algorithms effectively to solve moderately complex problems and optimize the resulting models. The course explores fundamental issues like data management, model selection, and complexity. Practical hands-on experience is emphasized, enabling participants to evaluate and report on the accuracy of models generated from real-world data. Enroll now to gain the necessary skills for successful machine learning implementation.
Learning Outcomes
- Have a good understanding of the fundamental issues and challenges of machine learning: data, model selection, model complexity, etc.
- Develop an appreciation for what is involved in learning models from data
- Understand a wide variety of learning algorithms
- Understand how to evaluate models generated from data
- Apply the algorithms to a real problem, optimize the models learned and report on the expected accuracy that can be achieved by applying the models
Course Benefits
- Practical hands-on machine learning experience.
- In-demand skills for the job market.
- Data-driven decision-making proficiency.
- Enhanced predictive modeling capabilities.
- Ability to automate complex tasks.
Employment Opportunities
- Data Scientist.
- Machine Learning Engineer.
- AI Specialist.
- Data Analyst.
- Research Scientist.
Industries Using Machine Learning
- Healthcare and Medicine.
- Finance and Banking.
- E-commerce and Retail.
- Manufacturing and Logistics.
- Technology and Software Development.
Pre-requisites
Essential Python programming knowledge is required.
System Requirements
Software: Any OS (winx, Linux, Mac)
- python3.x
- Anaconda 3 or newer.
Hardware: 8GB RAM
- 2GHz or faster processor (x86_64-compatible), multi-CPU configuration recommended
Who this course is for:
- Software engineers
- College Students
- Research scholar
- Anyone willing and interested to learn machine learning algorithms with Python
- Anyone who has a deep interest in the practical application of machine learning to real-world problems
Detailed course Content
Session – 1
- Introduction to Machine Learning
- Introduction to Machine Learning and Mathematical Preliminaries
- Purpose of machine learning
- Lifecycle of a machine learning model
- Learning Process
- Numpy
- Creating Arrays and Accessing Arrays
- Reshaping arrays
- Numpy operations (Random numbers, Matrix operations, Mathematical operations, Statistical Operations)
- Exercise on numpy
- Write a NumPy program to test element-wise for complex numbers, real numbers in a given array. Also, test if a given number is of a scalar type or not.
- Write a NumPy program to test whether two arrays are element-wise equal within a tolerance.
- Write a NumPy program to add a vector to each row of a given matrix.
- Write a NumPy program to create a two-dimensional array of a specified format.
- Write a NumPy program to create an NxN array. Create an array from said array by swapping first and last, second and third columns.
Session – 2
- Pandas – Series, DataFrame, Panel
- Pandas – Functionality, Reindexing and iteration
- Pandas – Indexing and selecting Data
- Pandas – Aggregations, Missing data, GroupBy, Categorical Data.
- Exercise on Pandas
- Write a Pandas program to create and display a DataFrame from a specified dictionary data which has the index labels.
- Write a Pandas program to select the rows where the score is missing, i.e. is NaN.
- Write a Pandas program to select the rows where number of attempts in the examination is based on conditions.
- Write a Pandas programs to perform grouping and Aggregating values
- Matplotlib – Plotting, Markers, Lines, Labels, Grid, Bars, Histograms
- Exercise on Matplotlib
- Matplotlib Legend Exercises
- Matplotlib subplot lib Exercise
- LineChar Exercise
- Scikit Learn – Introduction, Modelling Process, Data Representations
- Exercise on SciKit Learn
- Write a Python program to view basic statistical details like percentile, mean, std, etc. of iris data
- Write a Python program to get observations of each species (setosa, versicolor, virginica) from iris data.
- Write a Python program to draw a scatterplot, then add a joint density estimate to describe individual distributions on the same plot between Sepal length and Sepal width.
Session – 3
- Supervised Learning
- Regression analysis
- Linear Regression in Machine learning
- Hands-on – Stock Market Prediction, Market Sales Forecasting using existing data sets.
Session – 4
- Classification Algorithm
- KNN Algorithm
- Naive Bayes classifier
- Hands-on KNN Algorithm – generate train and test data using train_test_split of sklearn from iris data sets.
- Hands-on to Apply Naive Bayes using real data sets.