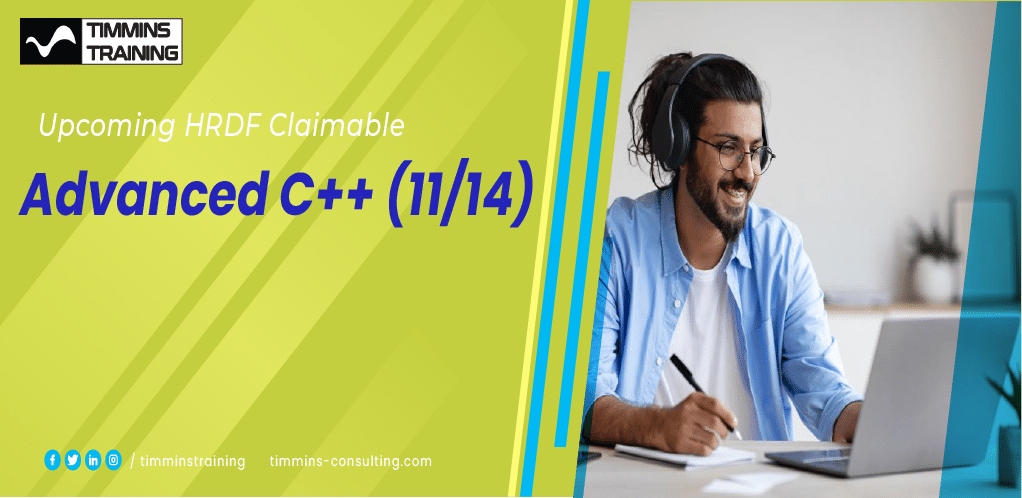
Modern C++ Programming (11/14)
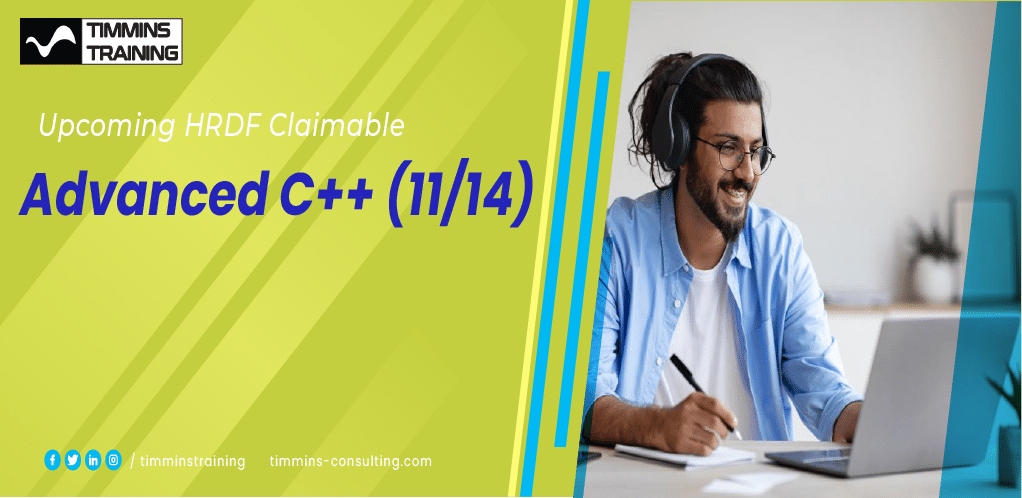
HRD Corp Course Registration No:
10001321003
Duration
16 hours
Description
Join our fast-paced introductory course to Modern C++. This versatile programming language, created by Bjarne Stroustrup, remains a popular choice for high-performance applications across diverse domains and platforms.
In 2011, C++11 introduced “Modern C++,” incorporating numerous new features like move semantics, automatic type inference, threading, lambda expressions, smart pointers, and more. C++14 followed, enhancing existing features and introducing additional capabilities.
Our course provides a solid foundation in Modern C++. Suitable for all skill levels, it equips you with the skills to leverage the language’s modern features effectively. Embrace the dynamic and versatile Modern C++ programming language by enrolling today!
Learning Outcomes
- Use C++ as an object-oriented language
- Demystify function & class templates
- Use STL components in your applications
- Write real-world applications in C++
- Apply Modern C++ (C++11/14) in Programs
Course Benefits of Learning C++ 11/14
- Enhanced programming capabilities and efficiency.
- Access to modern C++ features.
- Improved code readability and maintainability.
- Expanded career opportunities in software development.
- Ability to develop high-performance applications.
Employment Opportunities
- Software Engineer/Developer
- Systems Programmer
- Game Developer
- Embedded Systems Engineer
- Algorithm Developer
Industries Using C++
- Technology/Software Development
- Gaming and Entertainment
- Automotive and Transportation
- Financial Services
- Aerospace and Defense
Pre-requisites
Basic programming knowledge in any computer language
System Requirements
Software:
- Server OS: Any Linux distributions
- Compilers: g++ 4.x updated version ,
Debuggers:
- gdb
Hardware:
- 4GB memory minimum, 8GB recommended.
- 2GHz or faster processor (x86_64-compatible), multi-CPU configuration recommended.
Who this course is for
- College students who want to learn Modern C++
- C++ developers with varying levels of experience, who want to refresh the basics of the C++ language
- Software professionals who want to learn & implement Modern C++
- Anyone who is comfortable with other languages like C, Java, C#, Python, etc, and wants to learn & apply Modern C++
Detailed Course Content
Session 1: Introduction C++
- Compilation Steps
- Primitive Types and Variables
- Basic Input and Output
- Conditional and Looping statements
- Exercise on C++ operators, conditional test, and looping statements
- Functions
- Pointers, Reference
- Automatic type interface
- Exercise on pointers vs reference usages
Session 2: Class and Object Model
- Object Oriented Programming Features
- Class, object, Constructor, and Destructor
- Exercises on Employee enrollment application using class and object design style.
- this pointer
- static members
- Delegating Constructors(C++11)
- Default and Deleted Functions(C++ 11)
- Exercises in Constructors and destructors
- Exercise on Display Date using Constructors
Session 3: Overloading
- Operator Overload and Function Overloading
- Polymorphism, Virtual Function
- Exercise on to Perform Complex Operations using Overloading
- Exercise on demonstrating Order of constructor invocation
- Friend keyword, smart pointers
- Memory Management and Internals
- Exercise on demonstrate the working of friend function
- Exercise on to show shared ownership using smart pointers in C++.
- Create two classes C1 and C2 that share a common heap-based object d as data member.
Session 4: Templates
- Templates
- C++ 11 Template Features
- Lambda Expressions (C++ 11) and Internals
- C++ Concurrency
- Exercise on function objects and lambda expressions.
- Exercise on data shared between two threads.